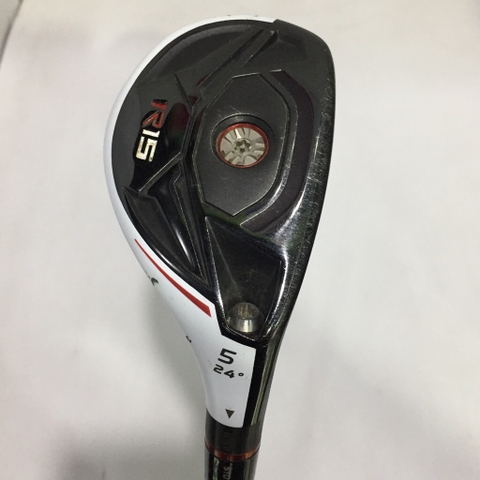
How do you use regex when golfing?
I can see three uses of regex when it comes to golfing: classic regex golf ("here is a list that should match, and here is a list that should fail"), using regex to solve computational problems and regular expressions used as parts of larger golfed code. Feel free to post tips addressing any or all of these.
What is regex?
Regex. Updated: 12/31/2020 by Computer Hope. Short for regular expression, a regex is a string of text that allows you to create patterns that help match, locate, and manage text. Perl is a great example of a programming language that utilizes regular expressions. However, its only one of the many places you can find regular expressions.
What is a regex in Perl?
Short for regular expression, a regex is a string of text that allows you to create patterns that help match, locate, and manage text. Perl is a great example of a programming language that utilizes regular expressions. However, its only one of the many places you can find regular expressions.
What are regular expressions and how to use them?
Regular expressions can also be used from the command line and in text editors to find text within a file. When first trying to understand regular expressions it seems as if it is a different language. However, mastering regular expressions can save you thousands of hours if you work with text or need to parse large amounts of data.

What does this regex do?
Regular expressions (shortened as "regex") are special strings representing a pattern to be matched in a search operation. They are an important tool in a wide variety of computing applications, from programming languages like Java and Perl, to text processing tools like grep, sed, and the text editor vim.
How do I learn regex?
Metacharacters allow you to write regular expression patterns that are even more compact....Metacharacters\d matches any digit that is the same as [0-9]\w matches any letter, digit and underscore character.\s matches a whitespace character — that is, a space or tab.\t matches a tab character only.
What is ?! In regex?
The ?! n quantifier matches any string that is not followed by a specific string n.
What is b regex?
The metacharacter \b is an anchor like the caret and the dollar sign. It matches at a position that is called a “word boundary”. This match is zero-length. There are three different positions that qualify as word boundaries: Before the first character in the string, if the first character is a word character.
Why is regex so hard?
Density. Regular expressions are dense. This makes them hard to read, but not in proportion to the information they carry. Certainly 100 characters of regular expression syntax is harder to read than 100 consecutive characters of ordinary prose or 100 characters of C code.
Is regex easy to learn?
Learning Regex is easier than you think. You can use this tool to easily learn, practice, test and share Regex.
What is regex example?
A simple example for a regular expression is a (literal) string. For example, the Hello World regex matches the "Hello World" string. . (dot) is another example for a regular expression. A dot matches any single character; it would match, for example, "a" or "1".
What is regex matches?
In regular expression syntax the period . mean "match any character" and the asterisk * means "any number of times". So it basically means match anything (even an empty string). It shouldn't filter out anything.
Is regex a language?
Regular Expressions are a particular kind of formal grammar used to parse strings and other textual information that are known as "Regular Languages" in formal language theory. They are not a programming language as such.
What does 1 mean in RegEx?
the first capturing groupThe backreference \1 (backslash one) references the first capturing group. \1 matches the exact same text that was matched by the first capturing group. The / before it is a literal character. It is simply the forward slash in the closing HTML tag that we are trying to match.
What is R RegEx?
The r means that the string is to be treated as a raw string, which means all escape codes will be ignored.
What does a zA Z0 9 mean?
The bracketed characters [a-zA-Z0-9] indicate that the characters being matched are all letters (regardless of case) and numbers. The * (asterisk) following the brackets indicates that the bracketed characters occur 0 or more times.
Is regex a programming language?
Regex has its own terminologies, conditions, and syntax; it is, in a sense, a mini programming language. Regex can be used to add, remove, isolate, and manipulate all kinds of text and data. It could be used as a simple text editor command, e.g., search and replace, or as it's own powerful text-processing language.
How can I learn regex in Java?
Example of Java Regular Expressionsimport java.util.regex.*;public class RegexExample1{public static void main(String args[]){//1st way.Pattern p = Pattern.compile(".s");//. represents single character.Matcher m = p.matcher("as");boolean b = m.matches();//2nd way.More items...
What is regular expression for dummies?
Regular Expression is a specially encoded language of character strings (i.e. [A-Z]+ or . *), used for matching simple or complex text patterns. Regular Expression can search, match, replace, substring, parse and split text. The latter three are functions mostly exploited in programming.
Is regex the same in all languages?
Regular expression synax varies slightly between languages but for the most part the details are the same. Some regex implementations support slightly different variations on how they process as well as what certain special character sequences mean.
xkcd
In the original xkcd strip, two examples of regex golf are given: the first, M | [TN]|B, is said to match the subtitles of all Star Wars films without matching any of the subtitles of Star Trek films, and the second, bu| [rn]t| [coy]e| [mtg]a|j|iso|n [hl]| [ae]d|lev|sh| [lnd]i| [po]o|ls, is said to match the last names of elected United States presidents but not their opponents; both regexes are analyzed by Explainxkcd.com and an analysis won't be repeated here..
Warmup
Warmup — Type a regex in the box. You get ten points per match (or lose ten, if you match something you shouldn't); each character costs one point.
Anchors
Anchors — You are deducted one point per character you use, and ten if you match something you shouldn't.
Ranges
Ranges — The test vectors were generated by grepping /usr/dict/words. Can you tell?
Backrefs
Backrefs — This doesn't really work as a tutorial. Not really clear what you're supposed to do here.
A man, a plan
A man, a plan — You're allowed to cheat a little. Even in hard mode, words will be no longer than 13 characters.
Prime
Prime — The length is not part of the string. I should probably have chosen a different color.
When using regex to solve computational problems or match highly non-regular languages, it is sometimes necessary to make
When using regex to solve computational problems or match highly non-regular languages, it is sometimes necessary to make a branch of the pattern fail regardless of where you are in the string. The naive approach is to use an empty negative lookahead:
Does regex have decimal characters?
Most regex flavours have predefined character classes. For example, d matches a decimal digit, which is three bytes shorter than [0-9]. Yes, they might be slightly different as d may also match Unicode digits as well in some flavours, but for most challenges this won't make a difference.
Does Ruby always match the beginning and end of a line?
Finally, Ruby has different semantics for line-related modifiers than most other flavours. The modifier that's usually called m in other flavours is always on in Ruby. So ^ and $ always match the beginning and end of a line not just the beginning and end of the string.
Is regular expression language agnostic?
There are a surprising amount of people who think that regular expressions are essentially language agnostic. However, there are actually quite substantial differences between flavours, and especially for code golf it's good to know a few of them, and their interesting features, so you can pick the best for each task. Here is an overview over several important flavours and what sets them apart from others. (This list can't really be complete, but let me know if I missed something really glaring.)
Can you adjust patterns in Python?
You can adjust the patterns and their order for the language you need to match. This technique works well for JSON, basic HTML, and numeric expressions. It has been used successfully many times with Python 2, but should be general enough to work in other environments.
Do you need to call to another group in regex?
There is no need to make the call to another (named or numbered) group inside that group itself. If you have a certain pattern that appears several times in your regex, just group it and refer to it outside that group. This is no different from a subroutine call in normal programming languages. So instead of.
Can you include a readable version of the code in addition to the competitive one?
For instance, answers to code-golf challenges should attempt to be as short as possible. You can always include a readable version of the code in addition to the competitive one. Explanations of your answer make it more interesting to read and are very much encouraged.
What is a regex in Perl?
Regex. Short for regular expression, a regex is a string of text that allows you to create patterns that help match, locate, and manage text. Perl is a great example of a programming language that utilizes regular expressions. However, its only one of the many places you can find regular expressions. Regular expressions can also be used ...
Where can I find regular expressions?
However, its only one of the many places you can find regular expressions. Regular expressions can also be used from the command line and in text editors to find text within a file. When first trying to understand regular expressions, it seems as if it's a different language.
